Let’s continue exploring C++23 features! Sometimes, accepted proposals introduce entirely new features, sometimes they bring bug fixes and sometimes they are somewhere in between.
The small feature we discuss today is from the latter category. Loops and conditionals in C++ have been evolving in a similar direction with the introduction of init statements. As we discussed in this article, since C++20 you can use a range-based for loop with an init statement:
1
2
3
for (auto strings = createStrings(); auto c: strings[0]) {
std::cout << c << " ";
}
What I missed in that earlier article is that we cannot only declare and initialize new variables in an init statement, but we can also use have typedef
if that’s what we need.
1
2
3
4
5
std::vector pointsScored {27, 41, 32, 23, 28};
for (typedef int Points; Points points : pointsScored) {
std::cout << "Jokic scored " << points << '\n';
}
You might run into a situation where scoping a typedef
for the for
-loop’s body is useful and this feature comes in handy. At the same time, if we need an alias, it’s more readable and also more widely usable to use using
.
Sadly, with C++20’s for
-loop init statements that’s not something you can do. You can use typedef
, but not using
.
Thanks to Jens Mauer’s paper P2360R0 this has changed with C++23. Alias declarations are also permitted now:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
#include <iostream>
#include <vector>
int main() {
std::vector pointsScored {27, 41, 32, 23, 28};
for (int points : pointsScored) {
std::cout << "Jokic scored " << points << '\n';
}
for (typedef int Points; Points points : pointsScored) {
std::cout << "Jokic scored " << points << '\n';
}
for (using Points = int; Points points : pointsScored) {
std::cout << "Jokic scored " << points << '\n';
}
}
Connect deeper
If you liked this article, please
- hit on the like button,
- subscribe to my newsletter
- and let’s connect on Twitter!
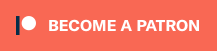